JavaFX Scoreboard v1.1 README
Overview
How to Run the Application
Command-line Switches
The Organization of the Souce Code
A Little more Detail on the
scoreboard.fx2.framework Package
Additional Notes
What's New
Overview
This
archive contains the source code required to build an electronic
scoreboard. It is written in Java utilizing the JavaFX
2.x API, and is packaged as a NetBeans
project. The type of scoreboard chosen for this
implementation pertains to ice hockey. With an
understanding of the overall organization and structure of the
code, it should be straightforward to extend scoreboard
functionality to include other sports.
At start up, the scoreboard
executes in one of two modes: as a master (the default), or as a slave. In master mode, the
scoreboard user interface is active. When a user moves
his/her pointing device over an editable part of the
scoreboard (a scoreboard digit), that component will, via
JavaFX animation, increase in size. This provides the
user a visual cue about what component is in focus. By
either clicking on the focused digit, or by utilizing
keyboard input, the user can change the value of the focused
digit. Each time a scoreboard digit is modified, an
XML packet is created describing the modification, and sent
out over an IP socket.
In slave
or remote scoreboard
mode, the scoreboard UI is inactive. That is to say,
it will not respond to any mouse or keyboard input.
Its display can only be updated by listening in on an
agreed-upon IP socket (configurable by command-line switch)
for XML scoreboard update packets. Upon receiving
those packets, the remote scoreboard instance will parse the
XML data and change the scoreboard display
accordingly. To start up a scoreboard in slave mode,
use the -slave command-line switch. It is
possible (and desirable) to have multiple slave scoreboards
simultaneously receiving updates from one master scoreboard.
How to Run the Application
The easiest way to run the scoreboard in either slave
or master mode is to utilize the pre-built Main
classes provided in the scoreboard.fx2 package
directory. Here's a partial list of those classes and
their meanings:
To see how a master scoreboard can
update a slave scoreboard, just start up the first of these two
main classes, Main and MainRemote on the same
system.
- Main.java - This will run the scoreboard in the
default (master) mode and open up an IP socket on the default
host (localhost) and port (2011). All scoreboard updates
will be sent over that defined socket.
- MainRemote.java - This will run an instance of a
slave or remote scoreboard. It will open up and listen in
on the default host (localhost) and port (2011) IP Socket. As
XML updates are received over the IP socket, the scoreboard
display will change accordingly.
The following main classes
demonstate different remote scoreboard clients
- MainRemoteClockOnly.java - It is possible to
configure a slave or remote client to display only a subset of
the master scoreboard's components. This remote or slave
instance of the scoreboard displays only the clock and listens
in on the default IP socket host and port.
- MainRemoteLED.java - The scoreboard source is
architected in such a way that with minimal work, an alternate
implementation of the look and feel can be implemented. In
this example, a remote scoreboard display has digits with an LED
segment look and feel. This display will listen in on the
default IP socket host and port.
The following main classes use
multicast sockets rather than IP sockets. In general, using multicast sockets
is not recommended as experience has shown, they tend to be
filtered out by all sorts of networking equipment.
- MainMulticast.java - Similar to Main.java,
this instance instead uses a default multicast socket and port
to communicate rather than a standard IP socket.
- MainMulticastRemote.java - The multicast socket
alternative to MainRemote.java.
Command-line
Switches
By running any of the main classes found in the scoreboard.fx2
package directory with the -help or --help
argument, the following description of command-line switches is
displayed to standard output. As we progress through this
document, the meaining of certain options will become more evident:
Command-line options:
-ConfigURL:URL (default: scoreboard/config/config.xml in Scoreboard.jar)
URL pointing to XML file describing remote client configuration
-DisplaySocket
Show socket connection info at bottom of scoreboard display
-DumpConfig:[true or false] (default false)
Dump layout of scoreboard in XML (for client customization)
-help or --help
Print this screen for command-line argument options and exit
-host:IP_ADDRESS (default: localhost)
Specify IP Address of socket
-master (default)
Run as a scoreboard controller (server)
-MulticastAddr:IP_ADDRESS (default: 227.27.27.27)
Use multicast socket and specify its IP address
-port:PORT_NUMBER (default 2011)
Specify port for socket connection
-slave
Run as a remote scoreboard client
-tv
Run in full screen mode for a TV (with padding for overscan)
-unlitOpacity:[0-100] (default 10)
Change opacity of unlit scoreboard bulbs
-UseIPSocket (default)
Use IP sockets (with defaults) for scoreboard updates
-UseMulticastSocket
Use multicast sockets (with defaults) for scoreboard updates
The
Organization of the Source Code
Here's a list of the relevant packages, and a brief description of
their contents
- scoreboard.common - Contains the constants,
interfaces and enums that are used throughout the source code
- scoreboard.config - Used to house sample XML
configuration files describing remote scoreboard layout and
configuration. There is no Java source in this directory,
only XML files.
- scoreboard.fx2 - This is where the various main Java
class files are located as described in the How to Run the Application section of
this document.
- scoreboard.fx2.framework - The heart of the
Scorebaord source code, this is where a great deal of the logic
for the scoreboard is contained. Many, but not all, of the
Java classes found in this package are abstract, and need to be
extended in order to create a real scoreboard
implementation. In particular, the look and feel of
scoreboard digits must be defined. Two such implementations
exist for this version of the code. They can be found in
the scoreboard.fx2.impl.bulb and scoreboard.fx2.impl.led
packages that follow.
- scoreboard.fx2.impl.bulb - In this implementation,
each scoreboard digit is comprised of 27 light bulbs that are
turned on and off to display the various numeric values.
It is meant to look like traditional light bulb based
scoreboards.
- scoreboard.fx2.impl.led - The scoreboard digits for
this implementation, are comprised of 7 LED segments that are
turned on and off to display various numeric values.
- scoreboard.fx2.framework.hockey - Contains the
extended classes that pertain to creating a scoreboard specific
to hockey. To create a scoreboard for a different sport,
it would make sense to start with these classes refactored over
to another package, for example, scoreboard.fx2.framework.basketball
- scoreboard.fx2.networking - This package contains the
JavaFX implementation of the abstract classes found in the scoreboard.common.networking
package. The reason for the abstraction layer here has to
do with the fact that UI platforms (like Swing and JavaFX)
require that work be done on the main thread. Each
platform achieves this in a slightly different way. In
JavaFX, the Platform.runLater() method has to be
utilized, while Swing, for example, requires use of the SwingUtilities.invokeLater()
method.
- scoreboard.fx2.util - Contains trivial utility class
specific to JavaFX
- scoreboard.util.sounds - Sample mp3 files that could
be used to customize the scoreboard horn sound
A Little More
Detail On the scoreboard.fx2.framework Package
The figure below represents a class hierarchy diagram of the
following packages:
- The abstract classes found in scoreboard.fx2.framework
- of which most are directly or indirectly derived from the
JavaFX base Parent class. In order to create an
alternate scoreboard implementation, the following lowest level
abstract classes must be extended: Digit, SingleDigit,
TwoDigit, Penalty, Clock and HockeyScoreboard.
The last class, HockeyScoreboard, could be replaced
with another abstract class (e.g. BasketballScoreboard)
that has a different look and behavior.
- The implementation classes found in scoreboard.fx2.impl.bulb
- These are the emboldend classes named with a "Bulb" prefix,
found at the bottom of the diagram. They represent the
classes used to implement scoreboard digits with a light bulb
look and feel.
- The implementation classes found in scoreboard.fx2.impl.led
- These are the emboldend classes named with an "LED" prefix,
found at the bottom of the diagram. They represent the
classes used to implement scoreboard digits with an LED segment
look and feel.
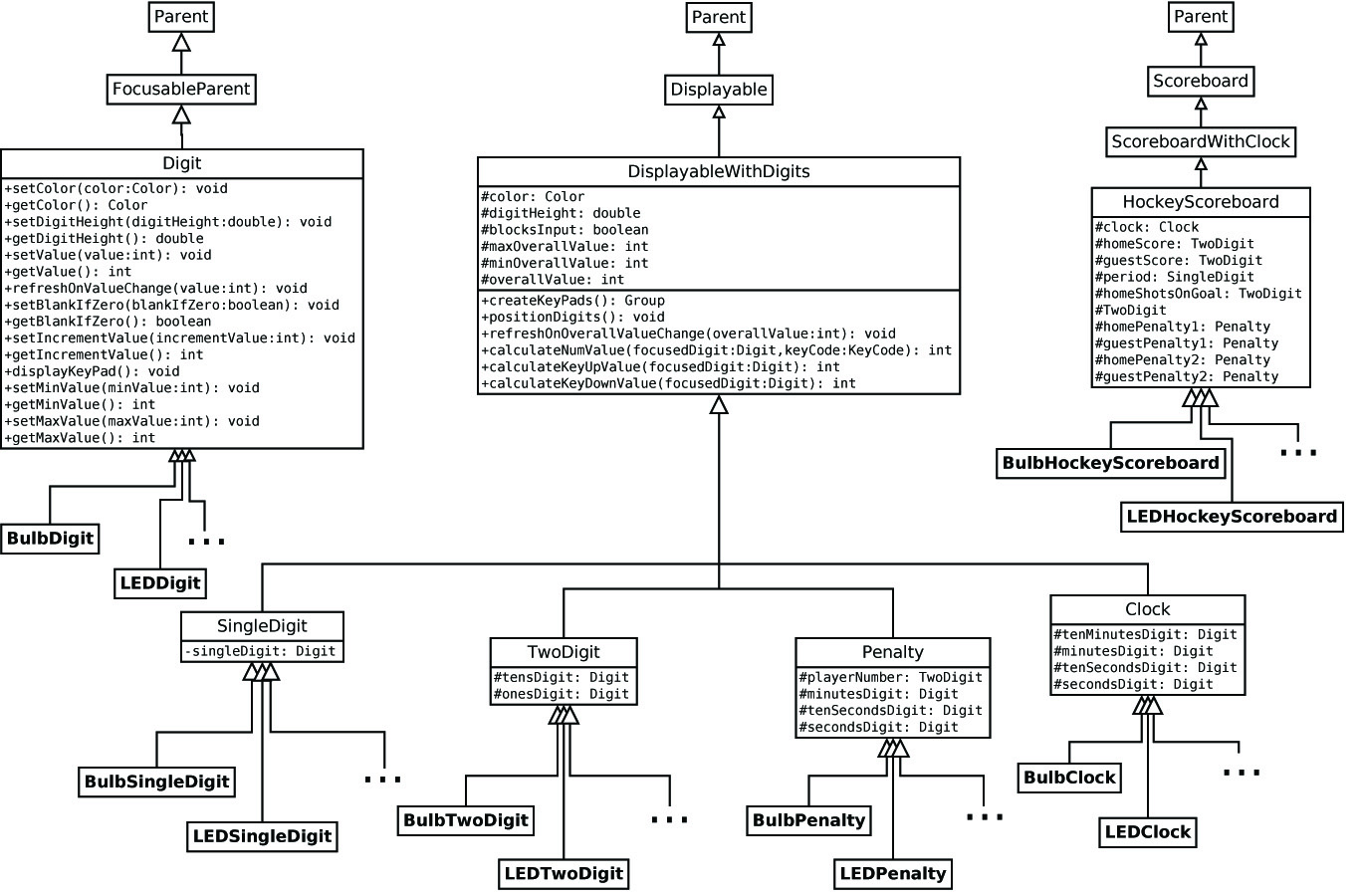
Additional
Notes
- There is some keyboard input support for this version of the
application. For example, the user can press the
<SPACE> bar to toggle starting and stopping the
clock. Additionally, the user can use the left and right
arrow keys to switch focus between digits of the same component.
- This source was derived originally from a project written in
the now defunct JavaFX Script. At the time of the original
writing, JavaFX Script was still in its early stages and the
work on layout managers was still in a state of flux.
Hence, the decision was made not to use any JavaFX LayoutManager
and instead rely on absolute coordinate positioning of
scoreboard components. The JavaFX 2.x version of the
Scoreboard application that exists here still has those
constraints. The unfortunate consequences are that a lot
of tedious positioning and sizing of components must take place.
- There are two sets of defined XML attributes used for this
application. One is used to describe and configure the layout of
a remote (slave) scoreboard client, where an informal
description of the specification can be found in the scoreboard.config.ConfigXMLSpec.xml
file. As referenced above, no JavaFX LayoutManager was used, so
some very primitive layout semantics are part of this
spec. The second set of XML attributes describe what a
scoreboard update looks like. A sample of possible
scoreboard update attributes can be found at scoreboard.config.UpdateXMLSpec.xml.
- The scoreboard.images directory/package contains
JPEG images of scoreboard digits. Not used for this
version of the source, the images could be used to
implement yet another version of the scoreboard that simply
displays an image associated with a digit value. A old
version of this application originally written in JavaFX Script
has an implementation with images, because it was much faster
than the rendered bulb and LED components. With JavaFX
2.x, performance is much improved and the need for image based
displays was no longer needed.
What's New
Version 1.1
Bug Fixes
- MainRemoteClockOnly.java bug. File URL referenced
originally pointed to file:///C:/Users/jtconnor... which
is local file not found in the source code. Fix points URL
to a config file in the Scoreboard.jar instead
- Bug displaying Penalty player number. If number ends in
0 (i.e. 10, 20, 30), that digit was blank.
Enhancements
- Fully implement Horn functionality, both local and remote.
- Manual horn button
- XML attributes for remote (slave) scoreboard horn
- Option for alternate horn sound - hornURL:URL
- Reorganize packages and code structure
- Separate out Globals, most go to scoreboard.common; the ones
specific to JavaFX go to scoreboard.fx2.framework
- Eliminate scoreboard.common.xml package. Move those
source files into scoreboard.fx2.framework and make them base
abstract classes
- Create a scoreboard.fx2.framework.hockey package containing
the implementation classes specific for a hockey
scoreboard. The classes extend ones found in
scoreboard.fx2.framework
- Eliminate scoreboard.common.networking.simple package and
move those classes into Test Packages.
- Global debugFlags variable with -debug:value command-line
switch
- Allow Penalty player numbers to have a zero prefix (i.e. "00",
"06" ...)